Implementing a Simple API
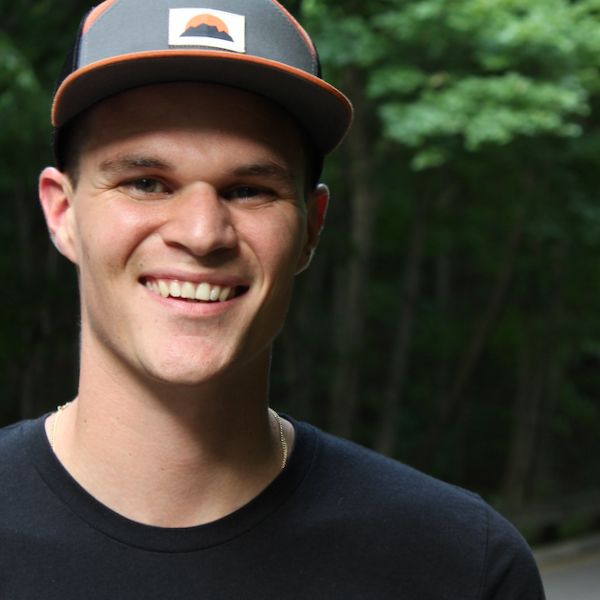
Colby Hemond
Implementing APIs doesn't have to be scary. Especially when you only GET data from them and not POST, PUT, or PATCH. Finding the right API to get started with might be the most challenging. Luckily there are some simple options out there. Like the Chuck Norris joke API, which I will be showing how I was able to quickly implement it.
There will be no frameworks or even linking to external script pages in this walkthrough—everything can be done right on your index.html
document. All you need to know are HTML, CSS, and some fundamentals of JavaScript.
We’ll go over:
- Reviewing the API and documentation
- Implementing the simplest version of the API
- Making it user-friendly by adding a button
- Implementing a dynamic API call
Reviewing the API and documentation
The first thing to do when beginning to implement an API is to review the documentation to gain an understanding of how it should be implemented and what functionalities it offers. It’s common for APIs to require a token or authorization. This one is free and read-only—no token needed.
You can test it quickly by pasting the URL below into your browser: https://api.chucknorris.io/jokes/random
Now let’s implement the simplest version of this API.
Implementing the simplest version of the API
First, create an element in your HTML where the joke will go:
<div class="quote-box">
<h3 id="quote"></h3>
</div>
Then add this JavaScript:
let quote = document.querySelector('#quote');
fetch('https://api.chucknorris.io/jokes/random')
.then(response => response.json())
.then(data => {
quote.innerHTML = data.value;
});
Now when the page loads, a Chuck Norris joke will be displayed!
Making it user friendly by adding a button
Let’s add a button:
<button id="new-chuck" onclick="getChuck()">Mr. Norris</button>
Update your JavaScript:
const getChuck = function () {
let quote = document.querySelector('#quote');
fetch('https://api.chucknorris.io/jokes/random')
.then(response => response.json())
.then(data => {
quote.innerHTML = data.value;
})
.catch();
};
getChuck(); // Load a joke on initial page load
Now clicking the button will load a new joke.
Implementing a dynamic API call
Let’s take it further and let users choose joke categories.
Add a dropdown:
<select id="chuck-categories"></select>
Then update your JavaScript:
const loadingText = function () {
let quote = document.querySelector('#quote');
quote.innerHTML = 'Wait for it...';
};
const setQuote = function (text) {
let quote = document.querySelector('#quote');
quote.innerHTML = text;
};
const setChuckCategories = function (categoriesArray) {
let categoriesSelect = document.querySelector('#chuck-categories');
categoriesSelect.innerHTML = categoriesArray
.map(category => `<option value='${category}'>${category}</option>`)
.join('');
};
const getSelectedChuckCategory = function () {
let category = document.querySelector('#chuck-categories');
if (category.options[category.selectedIndex] !== undefined) {
return category.options[category.selectedIndex].value;
} else {
return 'all';
}
};
const getChuck = function () {
loadingText();
const category = getSelectedChuckCategory();
let url = 'https://api.chucknorris.io/jokes/random';
if (category !== 'all') {
url += `?category=${category}`;
}
fetch(url)
.then(response => response.json())
.then(data => setQuote(data.value))
.catch();
};
const getChuckCategories = function () {
fetch('https://api.chucknorris.io/jokes/categories')
.then(response => response.json())
.then(data => {
data.unshift('all'); // add 'all' option
setChuckCategories(data);
})
.catch();
};
getChuck();
getChuckCategories();
Now your app will:
- Load categories into a dropdown
- Let users pick one
- Fetch a joke from that category on button click