Hello, Node!
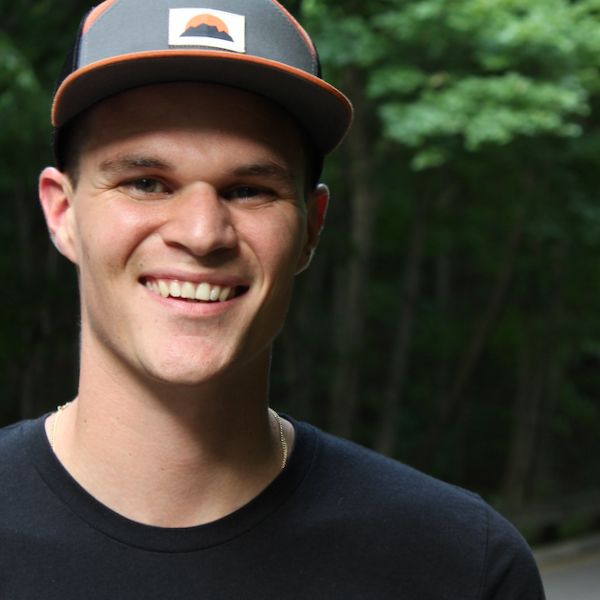
Colby Hemond
Move over Hello World, say wassup to Hello Node!
Our goal is to output "Hello Node!" to the terminal console by running the following command: node index.js
. Here are the steps we will go through to do this:
- Create a folder to hold our files
- Create the
index.js
file - Add content to the
index.js
file
Create a folder to hold our files
First things first, let's whip open that terminal. Make sure you are in a location that you want to create your folder. A quick list of commands to help us navigate the terminal:
ls
– list all files/folders in current directorycd /folder_name
– change directory into folderfolder_name
cd ../
– go back to the parent directory of the current directory
Creating our folder
Now we can start to use Node’s file system fs
while getting the project set up. While we’ll use this in the command line, we could also write these in a JS file.
Create a folder to hold our files:
node -e "fs.mkdirSync('hello_node')"
References:
Create the index.js
file
Navigating to the correct location
Use ls
to list the files in the directory—we should see the newly created hello_node
folder.
Move into the new folder:
cd hello_node
Creating the file
Create a new file index.js
:
node -e "fs.writeFileSync('index.js', '')"
This creates an empty file named index.js
.
Reference:
Add content to the index.js
file
Now add some content to the file using the same writeFileSync
method:
node -e "fs.writeFileSync('index.js', \"console.log('Hello, Node!')\")"
This writes console.log('Hello, Node!')
into the file.
Now execute the file from the terminal:
node index.js
Which outputs:Hello, Node!
What do we get out of doing this?
There are a few important fundamentals being practiced here. It’s not just about checking off a “getting started” project:
- Practicing terminal navigation
- Getting familiar with Node’s file system (
fs
) library - Executing Node commands in the terminal
Sure, you could right-click your way through a GUI or write node -e "console.log('Hello, Node!')"
for instant output—but we’re building muscle memory here.
Getting comfortable with these small steps helps you become fluent with Node workflows and CLI-based dev.